Ruby Classes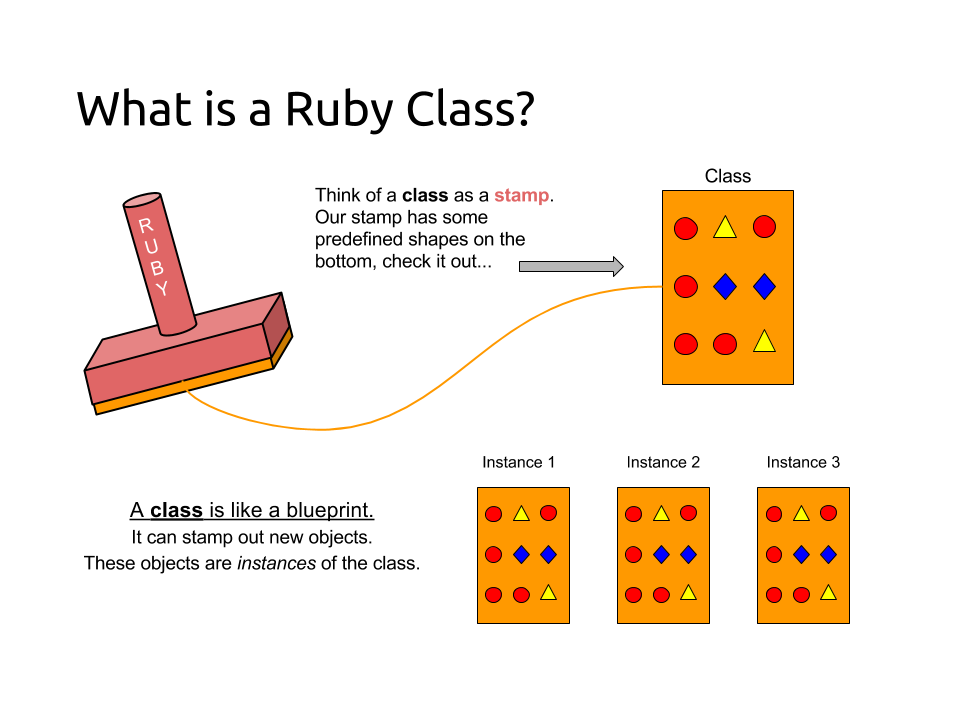
Ruby classes are blueprints for creating objects. Classes encapsulate functionality and data. A class is defined by the keyword "class" , followed by the class name. Every class will conclude with the keyword "end". The following is an example of an empty class:
class AdminUser
end
Notice how the naming convention for classes differs from that of variables. Class names begin with a capital letter and continue in PascalCase, capitalizing the first letter of each word. Although empty, our AdminUser constitutes a valid ruby class. We can now begin creating new instances of the class. These objects (instances) are created by calling a constructor, a special method associated with the class. The standard constructor is called "new".
Let's create our first object:
>> AdminUser.new
#<AdminUser:0x007fb2fbe40190>
We call the new constructor on the AdminUser class by using "dot notation". This is the syntax that ruby uses to send messages. Here we send the message "new" to the AdminUser class. Ruby responds by returning an object id, confirming that a new AdminUser object has been created.
Variables in a class
There are four types of variables provided by Ruby. Two types of variables you will use all of the time and two types you'll never use. We'll start by exploring the two you won't use.
$Global Variables are available across classes. The term "global" denotes the global, or application wide, namespace. Global variables are always preceded with a dollar ($) sign like $variable
.
@@Class Variables are available within a class, across different objects. They are always preceded by two at (@@) signs like @@variable
.
@Instance Variables are available across instances for any particular instance or object. They are always preceded by the at (@) sign like @variable
.
Local Variables are only available within the methods that define them. They begin with a lowercase letter or underscore (_) character, as in, variable
.
It is a bad practice to use global variables in almost every language. Global variables are available from anywhere in the code. This creates the opportunity for naming collisions if you are developing with gems or third party libraries. Two variables that share the same name but perform different functions can lead to unexpected behavior and errors that are hard to diagnose. A good practice is to limit your applications exposure to only what is necessary. The same goes for class variables. While there are use cases where they are necessary it is highly unlikely beginning developers will need them. For this reason they should be avoided. Instead, use local variables and instance variables when you need access to an objects data.
Inheritance
In OOP inheritance is a core concept that defines a relation between classes. In Ruby, a class inherits behavior from another class. The inheritor is said to be the child or subclass while the class it inherits from is called the parent or superclass. Let's look at an example:
class Animal
def speak
"Hello"
end
end
class Dog < Animal
end
fido = Dog.new # --> #<Dog:0x007fd76699edb0>
puts fido.speak # --> Hello
Inheritance is indicated by the less than " < " sign. Even though our Dog class doesn't have any methods defined, we can still call "speak" on our new Dog instance and it prints out "Hello". This is because the Dog class inherits the methods from Animal, which does have a speak method defined. Also, notice how we created a local variable, "fido", and assigned that variable to a new instance of the Dog class. Fido is now a bonafide Dog object that we can work with, as seen by our method call to speak. We've been talking about methods without officially defining them. This will be the subject of the next chapter. By this point, the relationship between classes and objects should be becoming clear. Remember when we created the AdminUser class? How did our AdminUser class know how to respond to "new"? The answer is inheritance. All classes inherit from Ruby's BasicObject class. This provides built-in functionality, including the "new" constructor.
Open/Close
Some programming languages have a concept where classes can be either opened or closed. Closed classes cannot be changed, nothing can be added or taken away. In Ruby, classes have no such distinctions. Classes are always open, available to be changed. You can even rewrite Ruby classes. This can be a source of great flexibility for advanced Ruby developers.
Single Inheritance
Ruby classes can inherit from at most one class. Ruby does not allow multiple inheritance. This is not restrictive as there are other ways to include functionality into classes. One way is by including modules, which are a topic of discussion later in this book.
Quick Summary: A class is a blueprint that can be used to stamp out new instances of an object.